Overview
The plugin is based on Twilio.
Twilio is a cloud communications platform as a service
(CPaaS) company based in
San Francisco, California
Twilio allows software developers to programmatically make
and receive phone
calls, send and receive text
messages, and perform other communication functions using its web service APIs.
Get started
To use the plugin you must have a Twilio account.
If you already have Twilio account you can skip this step and start with section
Configure Twilio
# Create a Twilio account
How to Work with your Free Twilio Trial Account
Follow the link above and complete only the first three steps.
- Sign up for your free Twilio trial
- Verify your personal phone number
- Get your first Twilio phone number
# Configure Twilio
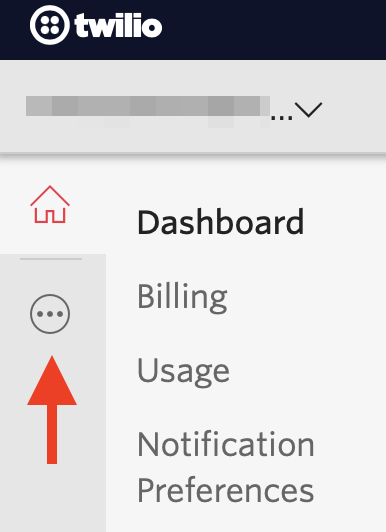
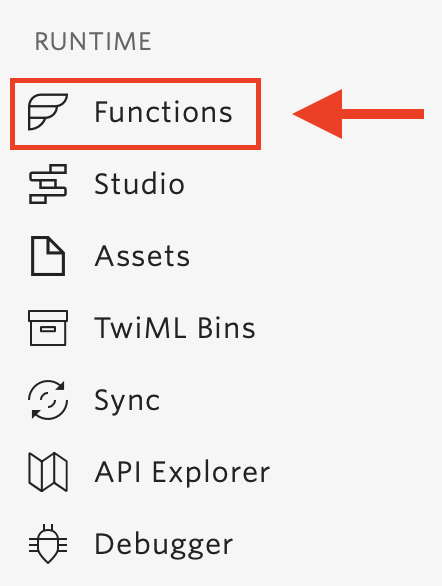
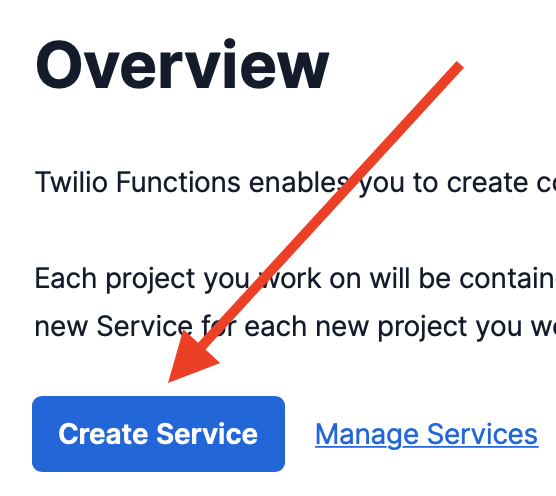
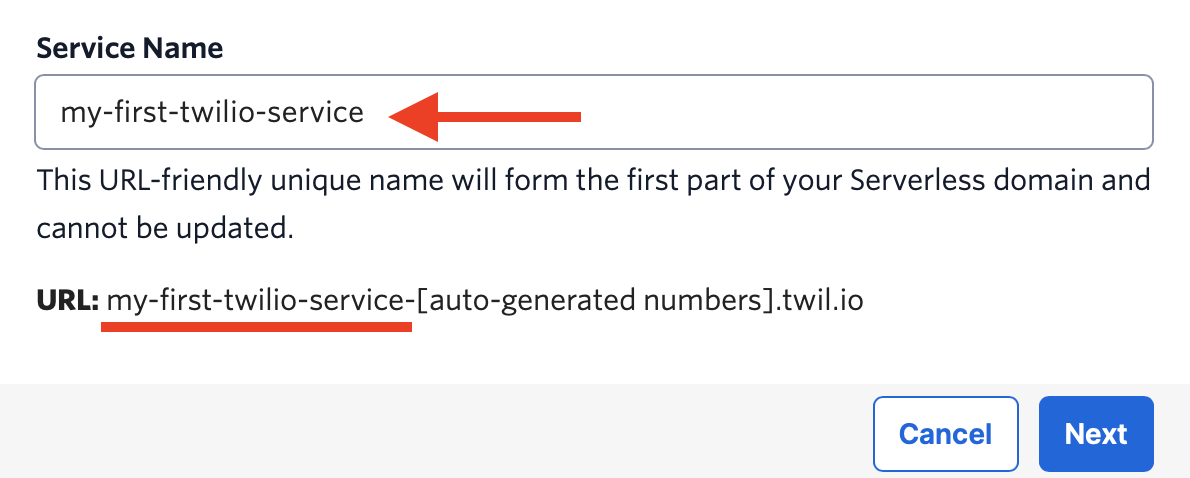
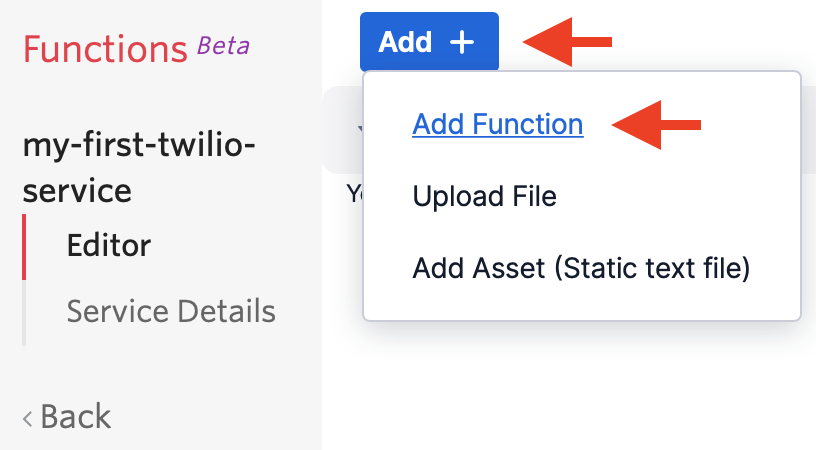
Create 3 functions with the following paths
/token
/phone-numbers
/call
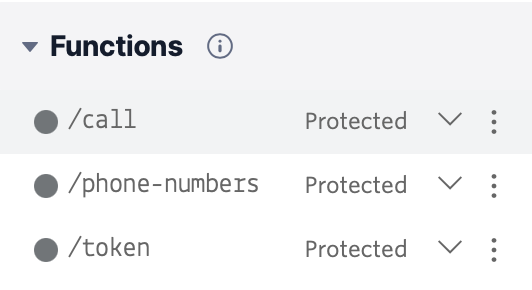
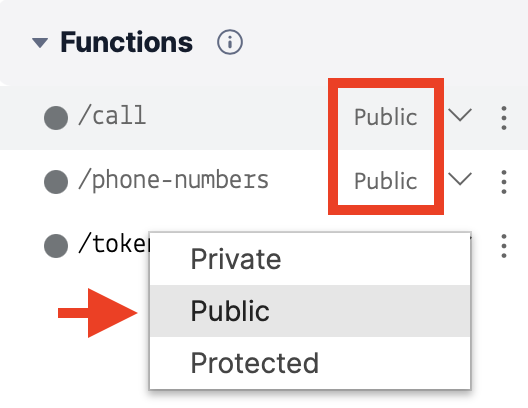
In each created function, you must insert the corresponding program code.
Inside ZIP Archive you downloaded from CodeCanyon you will find a Twilio Functions folder with three different text files:
token.txt
phone-numbers.txt
call.txt
The contents of these files must be inserted into the corresponding functions and for each function, save by clicking the Save button.
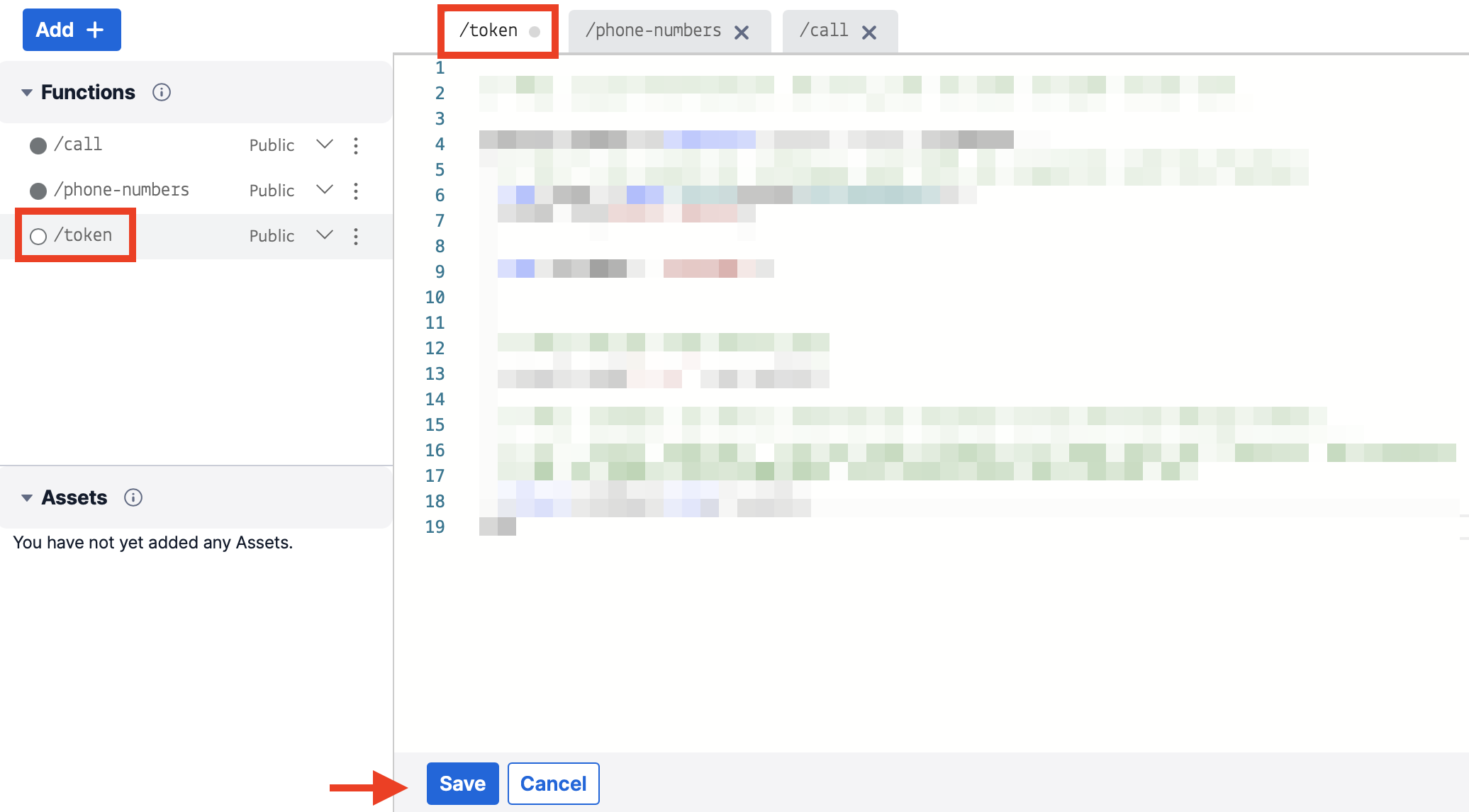
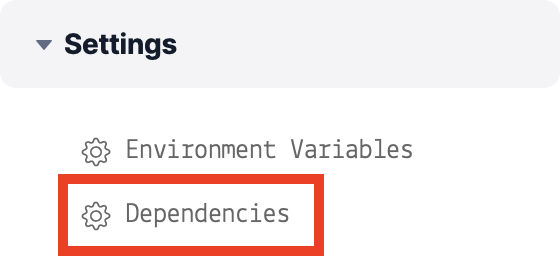

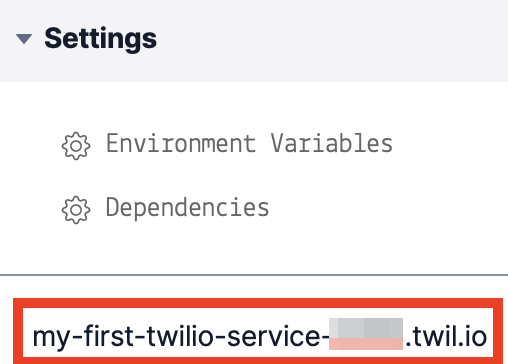
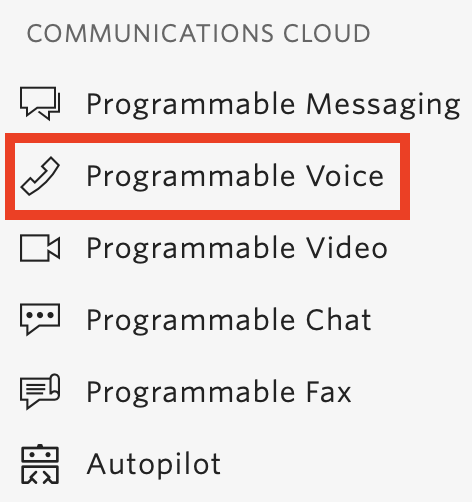
Next, go to the TwiML Section.
Find and open the TwiML Apps subsection and click on the Create new TwiML App button
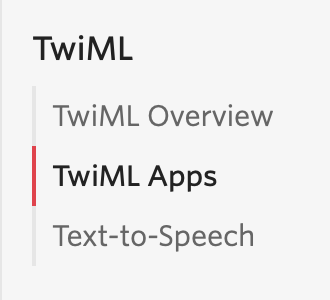
Name the TwiML App InTouchWebDialer.
Next, find the Voice block and in the REQUEST URL field, insert the domain name of the service that Twilio created for us. See step 11
Make sure the URL starts with https:// and ends with /call
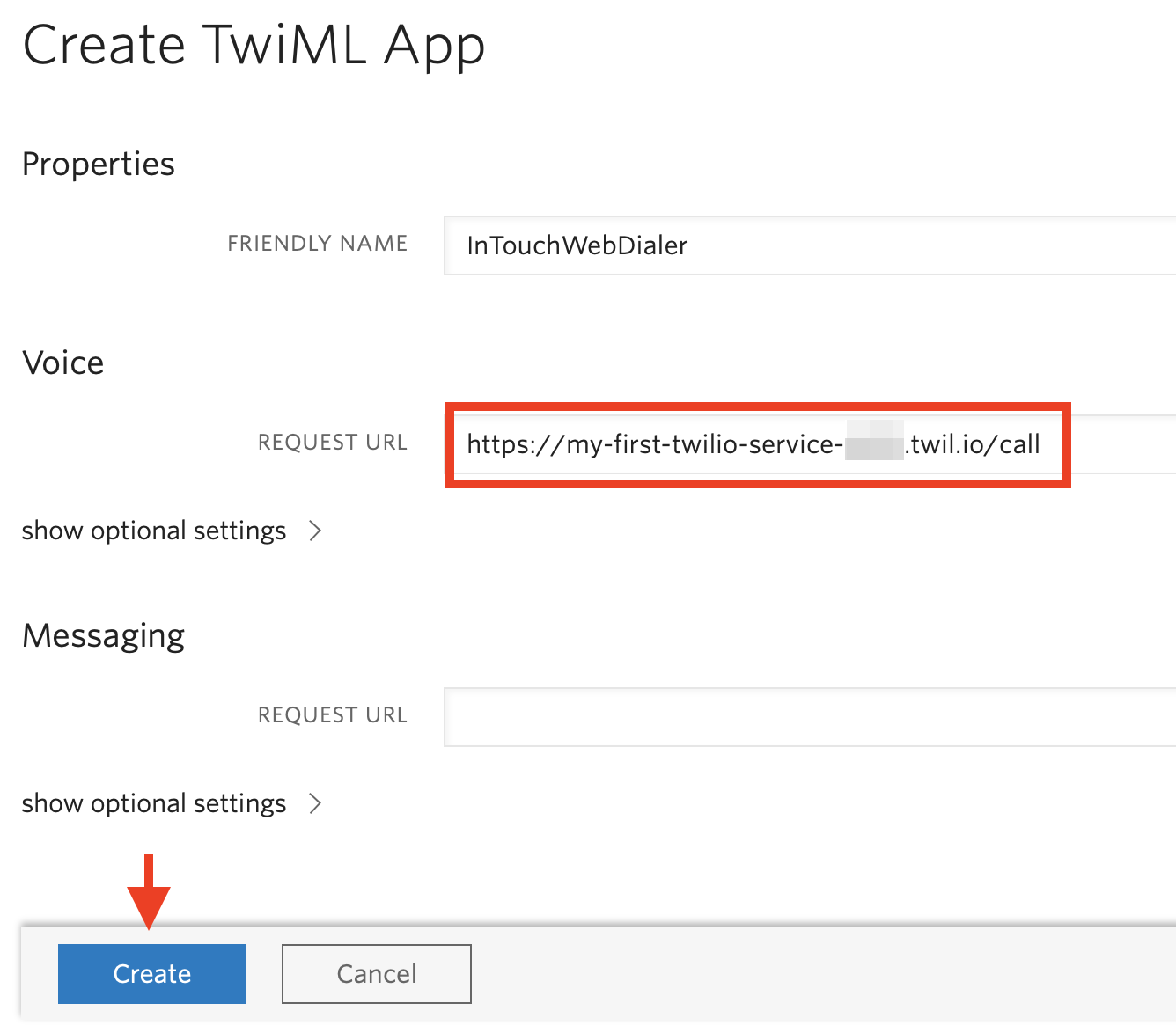
After we have created our new TwiML app, we need to find and copy its unique SID.
To do this, find the newly created app in the list of all TwiML APPs and open it.

Copy the SID to the clipboard
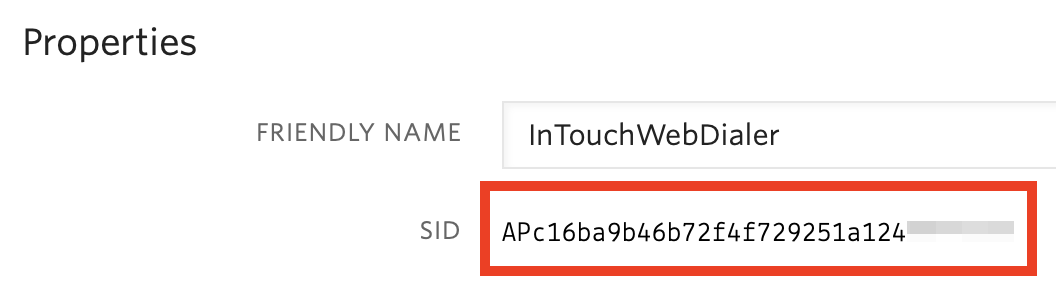
Go back to Functions → Services
https://www.twilio.com/console/functions/overview/services
Open the new service we just created and find the Settings section and navigate to the Environment Variables section.
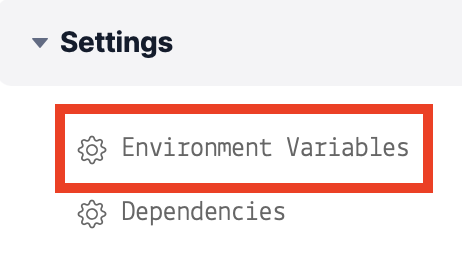
Make sure the option Add my Twilio Credentials (ACCOUNT_SID) and (AUTH_TOKEN) to ENVchecked.
You also need to add a new variable TWIML_APP_SID with the value we copied in step #15.
Next, click on the Deploy All button.
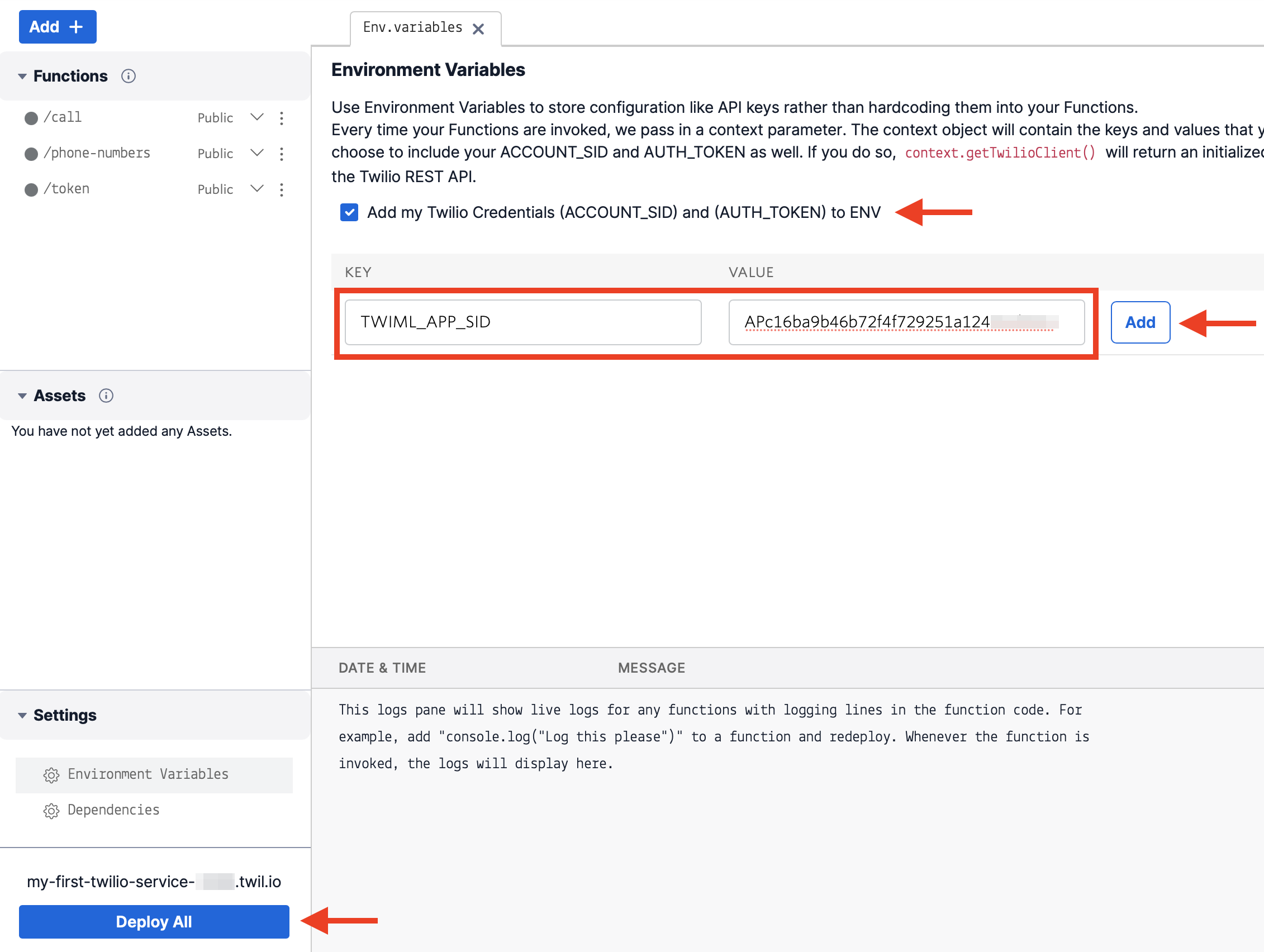
After a successful deployment, you should see something like this in the console.
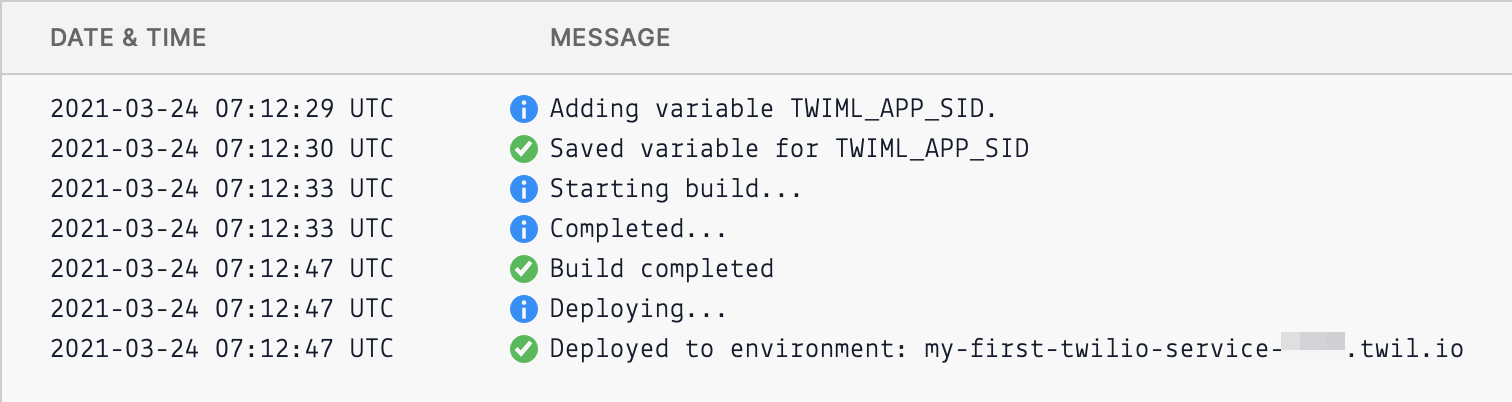
Next, you need to apply the settings to your phone numbers.
To do this, go to the Active Numbers section.
https://www.twilio.com/console/phone-numbers/incoming
Open the number that you want to associate with our service.
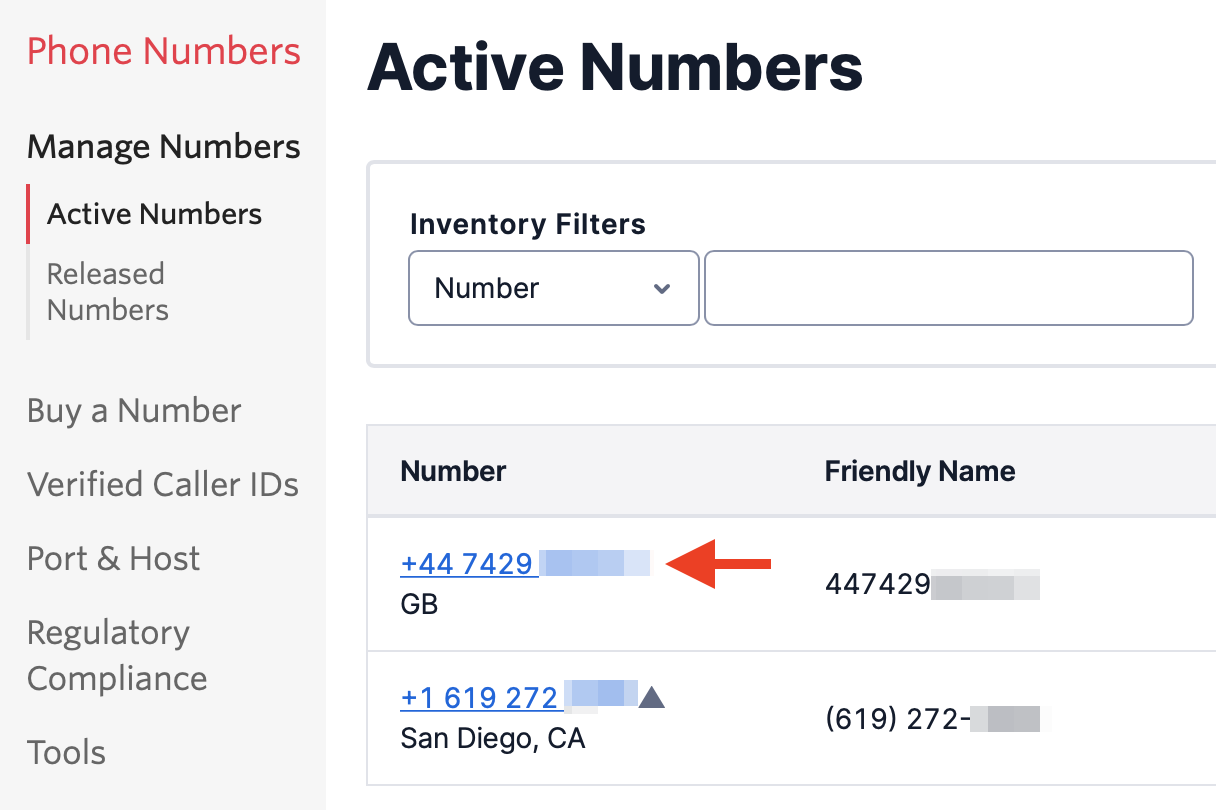
Find the Voice & Fax block.
CONFIGURE WITH should be TwiML app.
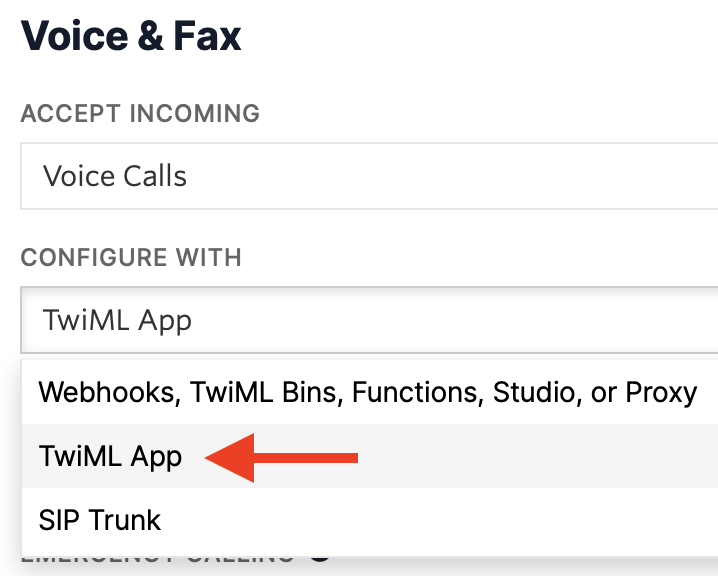
In the TWIML APP drop-down list, select InTouch TwiML APP
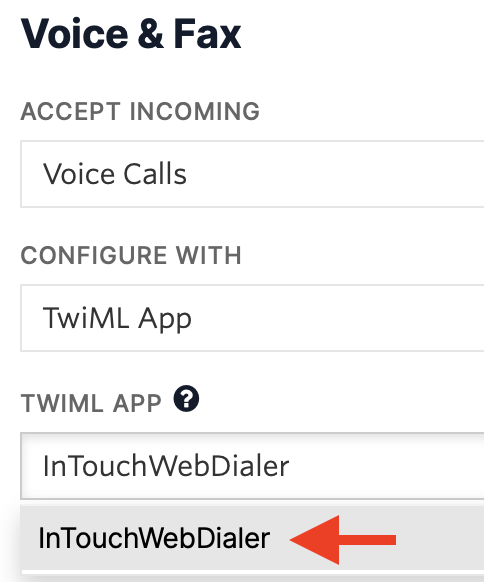
Click Save
This procedure must be done with all the phone numbers that you want to associate with the plugin.
Plugin Installation
After a successful purchase, you will be able to download ZIP archive.
In this archive you will find a js folder with 2 javascript files.
iframe-transport.js
intouch-web-dialer.js
iframe-transport.js
This file provides two-way communication between your web page and the embedded frame, in which the telephony plugin will be located.
intouch-web-dialer.js
This file contains the user interface, all the logic for making outgoing calls, as well as for receiving calls.
Below is an example of how to connect a plugin to an empty html page
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div id="intouch-iframe-transport"></div>
<script src="https://site.com/js/iframe-transport.js"></script>
<script type="text/javascript">
document.addEventListener("DOMContentLoaded", function (event) {
IntouchTransport.init("https://site.com/js/intouch-web-dialer.js", {
"baseUrl": "https://intouch-web-dialer-[generated-id].twil.io"
});
});
</script>
</body>
</html>
Usage
# Methods
IntouchTransport.init(path: String, settings: Object)
- path should contain absolute path to the intouch-web-dialer.js file.
- settings it is an object of keys and their values. See the Props section below.
Examples:
IntouchTransport.init("https://site.com/js/intouch-web-dialer.js", {
baseUrl: "https://intouch-web-dialer-[generated-id].twil.io"
});
IntouchTransport.init("https://site.com/js/intouch-web-dialer.js", {
baseUrl: "https://intouch-web-dialer-[generated-id].twil.io",
allowIncomingCalls: true,
allowOutgoingCalls: true,"
showDialPad: true,
canMute: false,
'input.onlyCountries': ["US", "CA", "AU"],
'input.disabledFetchingCountry': true,
'input.preferredCountries': ['US']
});
IntouchTransport.configure(key: String, value: Object)
IntouchTransport.configure('allowIncomingCalls', true);
IntouchTransport.configure('input.preferredCountries', ["US", "CA"]);
You cannot use following keys in the .configure() method.
- baseUrl
- allowIncomingCalls
- allowOutgoingCalls
These keys should be specified only in the .init() method.
IntouchTransport.setNumber(phoneNumber: String)
IntouchTransport.setNumber('+447429995617');
This method will set the phone number passed as an argument and perform validation.
IntouchTransport.setNumberAndCall(phoneNumber: String)
IntouchTransport.setNumberAndCall('+16192722992');
This method will set the phone number passed as an argument and perform validation.
If the validation is successful, the web plugin initiates an outgoing call.
# Props
PARAMETER | DESCRIPTION |
---|---|
baseUrl required | baseUrl should contain absolute path to the intouch-web-dialer.js file. |
allowIncomingCalls optional default: true | By default, the plugin can accept incoming calls. If you set this value to true, the plugin will no longer receive incoming calls. |
allowOutgoingCalls optional default: true | By default, the plugin can make outgoing calls. If you set this value to true, the plugin will no longer make outgoing calls. |
showDialPad optional default: true | By default, the plugin displays dialpad. If you set this option to false - the dialpad will no longer be displayed |
canMute optional default: true | When this option is set to false, you cannot use the Mute/Unmute button. |
# Events (Coming soon ...)
Others
# Bug reporting (Coming soon ...)
# Feature requests (Coming soon ...)
# Credits
Credits
- Making and receiving voice calls to and from a web browser by twilio-client.js.
- Telephone Number parsing, validation by vue-tel-input.
- Country Codes data from intl-tel-input.
- User's country by ip2c.org, request using Fetch API.